AJAX technology (AJAX = Asynchronous JavaScript And XML) is a developer’s dream because it can be used to update the content of a web page without reloading the whole page. As you know it is possible to update parts of a web page without refresh the whole page or reloading the page. In conventional web applications data from the server and data to the server are sending synchronously. Which means that when we hit the submit button, it will direct to a new page with new content. By using AJAX we can update the part of a web page by sending the data to the server or retrieve data from the server asynchronously. Web applications can send and retrieve data from server asynchronously without affecting the display behavior and interfering the current content of the web page.
This tutorial is going to discuss about the usage of AJAX in PHP. The sample PHP code to populate districts based on state name in the drop down list using AJAX without refreshing the whole page. AJAX is a browser based technology which is independent of server technology. Mouse click event is sufficient to update the portion of content in web applications.
Sample PHP code to populate districts based on state name using AJAX
AJAX uses XMLHttpRequest object (browser built in object). The main part of AJAX is the XMLHttpRequest object. This object is used to request data from the server. The results are manipulated using javascript and results are displayed using HTML.
There are two ways of creating AJAX object. First method of creating AJAX object is
var xhttp = new XMLHttpRequest();
This method of object creation is not supported in older version of browsers. In order to avoid the errors ,the professional way (second method) of creating AJAX object is shown below. The getXMLHTTP() method will return the AJAX object in req and based on that object we can load the districts up on selection of state.
var req = getXMLHTTP(); if (req) { // code here } function getXMLHTTP() { var xmlhttp=false; try { xmlhttp=new XMLHttpRequest(); } catch(e) { try { xmlhttp= new ActiveXObject("Microsoft.XMLHTTP"); } catch(e) { try { xmlhttp = new ActiveXObject("Msxml2.XMLHTTP"); } catch(e1) { xmlhttp=false; } } } return xmlhttp; }
Working of sample PHP code to select a state, the corresponding districts will load in the drop down list Using AJAX is as follows. The three sample PHP code to populate districts based on state name in the drop down list using AJAX are dbconnection.php, index.php, finddistrict.php. These samples codes you should keep in your root directory of PHP (www folder).
Step 1: Database connection code in PHP
Create a database connection code separately for importing database connection wherever necessary. So that we can avoid of inserting the db connection code in each and every page.
‘dbconnection.php’
<?php $servername = "localhost"; $username = "root"; $password = "admin123"; $dbname = "cme"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("Connection failed to MySql " . $conn->connect_error); } /* else { echo "MySql Server Opened...\n"; } */ ?>
Step 2 : User Registration form
Create a User registration form as shown in the figure. There is two drop down list in the registration form. First drop down list is used to select a state ,based on that state the corresponding districts will list in the second drop down list.

The code for creating the user registration form as shown in the above figure is
Index.php
<?php require_once('dbconnection.php'); ?> <html> <head> <title>Registration</title> </head> <body> <form action="user_create.php" method="post"> h2> Registration Form</h2> <input type="text" name="firstname" id="firstname" placeholder="First name"><br><br> <input type="text" name="lastname" id="lastname" placeholder="Last name"><br><br> <input type="email" name="email_id" id="email_id" placeholder="Email"><br><br> <input type="text" name="username" id="username" placeholder="Userrname"><br><br> <input type="password" name="password" id="password" placeholder="Password"><br><br> <input type="password" name="rpassword" id="rpassword" placeholder="Repeat Password"><br><br> <?php $sql1 = "SELECT * FROM state"; $rs1 = mysqli_query($conn, $sql1); ?> <select STYLE='font-weight: bold; font-size: 15px;' name="state" id="state" onChange="find_district();"> <option>Select State </option> <?php while($row1 = mysqli_fetch_array($rs1, MYSQLI_ASSOC)) { ?> <option value=<?php echo $row1['id']?>><?php echo $row1['statename']?></option> <?php } mysqli_close($conn); ?> </select> <br> <br> <select STYLE='font-weight: bold; font-size: 15px;' name="district" id="district" > <option>Select district </option> </select> <br> <br> <input type="submit" name="register" value="Register Now" class="btn-login"> </body> </html> <script type="text/javascript"> function find_district() { var stateid=document.getElementById("state").value; var countryid=1; var strURL="finddistrict.php?country="+countryid+"&state="+stateid; var req = getXMLHTTP(); if (req) { req.onreadystatechange = function() { if (req.readyState == 4) { if (req.status == 200) { document.getElementById('district').innerHTML=req.responseText; } else { alert("Problem while using XMLHTTP:\n" + req.statusText); } } } req.open("GET", strURL, true); req.send(null); } } function getXMLHTTP() { var xmlhttp=false; try { xmlhttp=new XMLHttpRequest(); } catch(e) { try { xmlhttp= new ActiveXObject("Microsoft.XMLHTTP"); } catch(e) { try { xmlhttp = new ActiveXObject("Msxml2.XMLHTTP"); } catch(e1) { xmlhttp=false; } } } return xmlhttp; } </script>
Before we proceed create a database name as cme and table name as state as shown below.In the above code as you can see that, database connection has been established and created two drop down list one for loading the state and the other for loading the district. Initially we need to load the states from the database in the first drop down list.
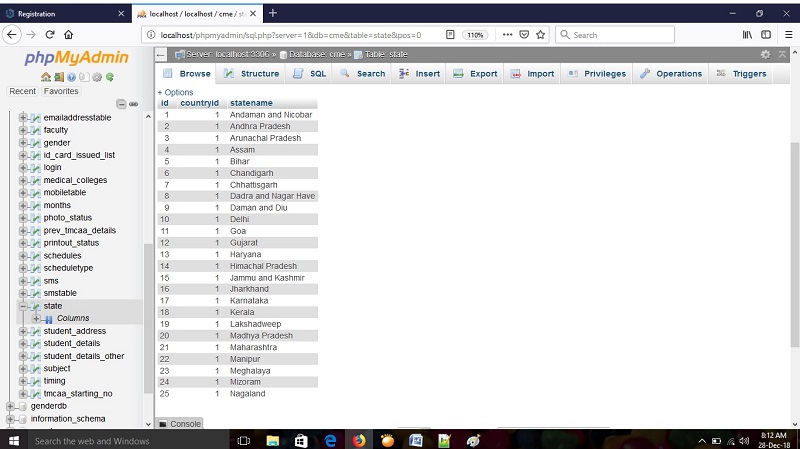
Our aim is to discuss Sample PHP code to select a state, the corresponding districts will load in the drop down list Using AJAX. Create a table district in the database as shown below.
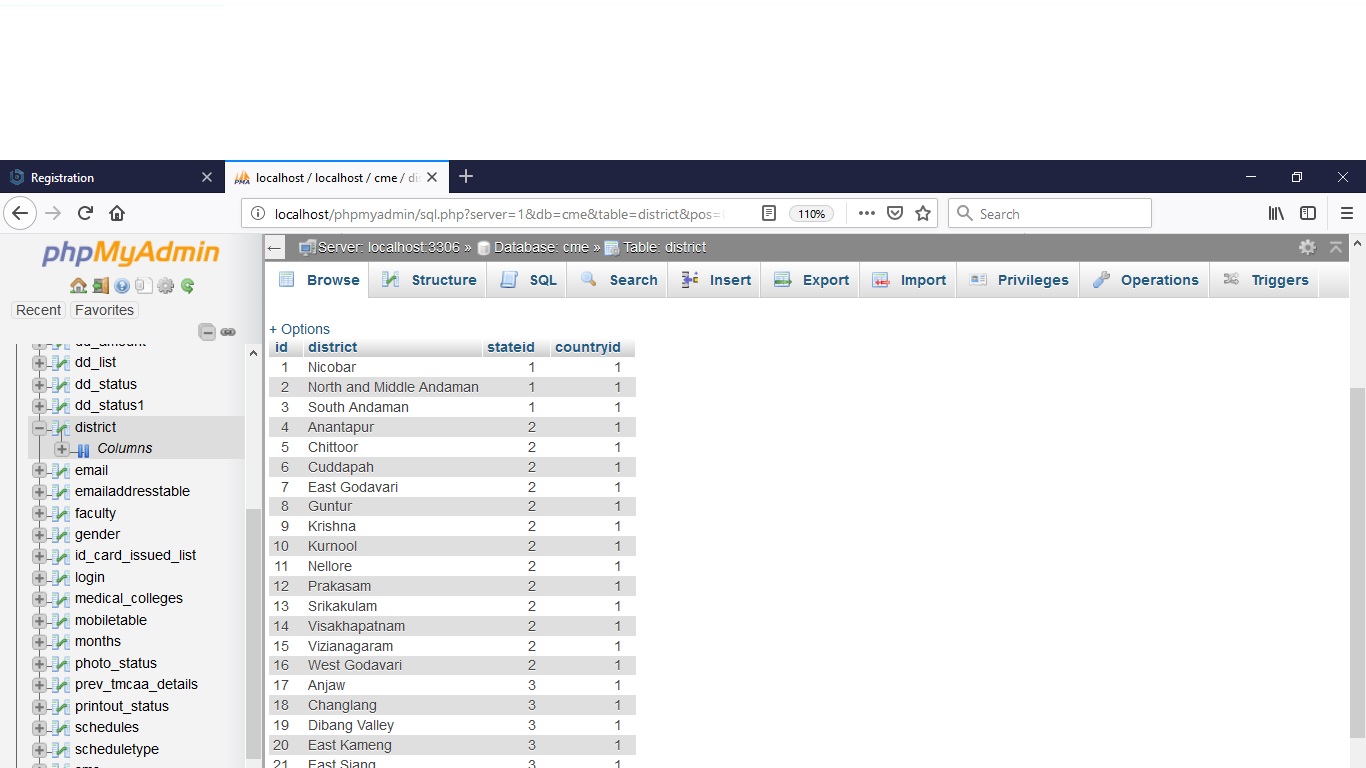
The below code is to load the states from the database in the first drop down list which is already specified in the index.php page.
<?php $sql1 = "SELECT * FROM state"; $rs1 = mysqli_query($conn, $sql1); ?> <select STYLE='font-weight: bold; font-size: 15px;' name="state" id="state" onChange="find_district();"> <option>Select State </option> <?php while($row1 = mysqli_fetch_array($rs1, MYSQLI_ASSOC)) { ?> <option value=<?php echo $row1['id']?>><?php echo $row1['statename']?></option> <?php } mysqli_close($conn); ?> </select>
When a state is selected from the first drop down list an onChange event fires, it calls the event handler function “find_district()” . This function is to load the corresponding districts based on the selection of state in the first drop down list.
Step 3 : Loading districts based on state selection
As we already discussed to load the district names based on state name we need to create a separate page named as finddistrict.php. In this page create a drop down list to load the district names. The finddistrict.php page should contain a drop down list which have the name and its id same as in the drop down list created for loading the district in the user registration form (index.php). The code below showing a drop down list to load the districts from the user registration form that we already created above.
<select STYLE='font-weight: bold; font-size: 15px;' name="district" id="district" > <option>Select district </option> </select>
When we select a state from the first drop down list in the user registration form,the onChange event fires. The event handler for onChange event will call the “find_district()” function.The find_district() will redirect the state Id and country id of selected state name to the page finddistrict.php for loading the districts. The districts are loaded based on state id using the page finddistrict.php and results are redirected to the second drop down list of user registration form.
finddistrict.php
<?php require_once('dbconnection1.php'); $countryId=intval($_GET['country']); $stateId=intval($_GET['state']); $sql1 = "SELECT id,district FROM district WHERE countryid='$countryId' AND stateid='$stateId'"; $rs1 = mysqli_query($conn, $sql1); ?> <select STYLE='font-weight: bold; font-size: 15px;' name="district" id="district" > <option>Select District </option> <?php while($row1 = mysqli_fetch_array($rs1, MYSQLI_ASSOC)) { ?> <option value=<?php echo $row1['id']?>><?php echo $row1['district']?></option> <?php } mysqli_close($conn); ?> </select>
One Reply to “Sample PHP code to populate districts based on state name using AJAX”