How to Add Two Numbers in Android
In this tutorial, I have created a simple android application for adding two numbers. It is a simple beginner’s level application. Once you understood this simple android application for adding two numbers you can implement the other features of a basic calculator.
Start a new project in Android Studio using an Empty Activity. Here I just named my android application name as Sum of Two Numbers. Once the project has been created, you can see the basic android project structure.
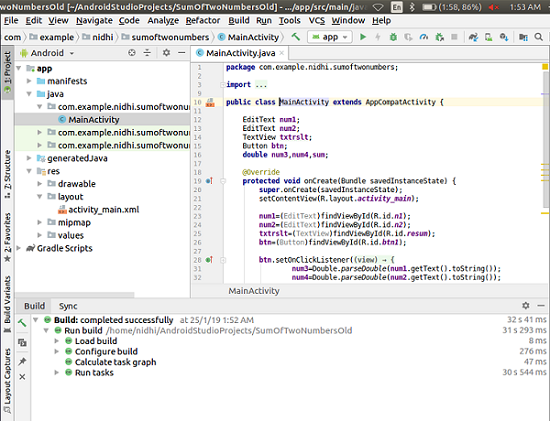
First you create a layout for input two numbers and a Button. When you click on the button sum will be displayed in another activity. I have created two TextView ( TextView control in android displays text to the user) , two EditText ( a user interface of entering text and modifying) and a Button for submitting the values.
Layout for adding Two Numbers
Modify your activity_main.xml file as shown below by open up the xml file in res/layout.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.nidhi.sumoftwonumbers.MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="100dp" android:id="@+id/l1" > <TextView android:id="@+id/maintxt" android:layout_width="match_parent" android:layout_height="match_parent" android:text="Addition" android:textSize="25sp" android:textAlignment="center" /> </LinearLayout> <LinearLayout android:id="@+id/l2" android:layout_width="match_parent" android:layout_height="100dp" app:layout_constraintTop_toBottomOf="@id/l1" tools:layout_editor_absoluteX="0dp"> <TextView android:id="@+id/text1" android:layout_width="wrap_content" android:layout_height="39dp" android:text="Enter First Number" /> <EditText android:id="@+id/n1" android:layout_width="50dp" android:layout_height="50dp" android:layout_alignRight="@+id/text1" android:layout_marginLeft="100dp" /> </LinearLayout> <LinearLayout android:id="@+id/l3" android:layout_width="match_parent" android:layout_height="140dp" app:layout_constraintTop_toBottomOf="@id/l2" tools:layout_editor_absoluteX="0dp"> <TextView android:id="@+id/text2" android:layout_width="wrap_content" android:layout_height="match_parent" android:text="Enter Second Number" /> <EditText android:id="@+id/n2" android:layout_width="50dp" android:layout_height="50dp" android:layout_alignRight="@+id/text2" android:layout_marginLeft="100dp" /> </LinearLayout> <LinearLayout android:id="@+id/l4" android:layout_width="match_parent" android:layout_height="151dp" android:layout_marginBottom="3dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@id/l3" app:layout_constraintVertical_bias="1.0"> <Button android:id="@+id/btn1" android:layout_width="73dp" android:layout_height="50dp" android:layout_marginLeft="100dp" android:text="SUM" /> <TextView android:id="@+id/resum" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="150dp" /> </LinearLayout> </android.support.constraint.ConstraintLayout>
The layout will look like this.
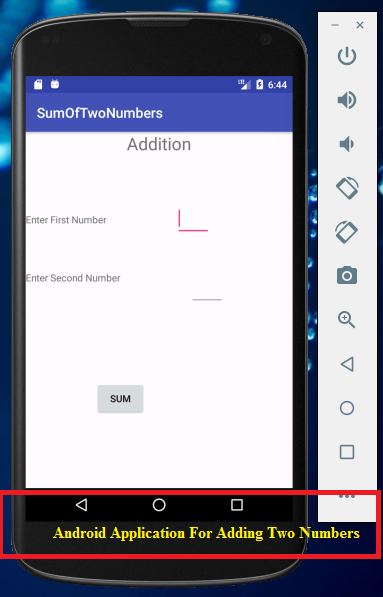
Adding Two Numbers
When the sum button has been clicked , we need to calculate sum of values entered by the user in the text boxes, sum it up and render the output in the second activity.
Modify your MainActivity.java file as follows.
MainActivity.java
package com.example.nidhi.sumoftwonumbers; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends AppCompatActivity { public static final String Send_Result=""; //"com.example.nidhi.SumOfTwoNumbers"; EditText num1; EditText num2; TextView txtrslt; Button btn; double num3,num4,sum; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); num1=(EditText)findViewById(R.id.n1); num2=(EditText)findViewById(R.id.n2); txtrslt=(TextView)findViewById(R.id.resum); btn=(Button)findViewById(R.id.btn1); btn.setOnClickListener(new OnClickListener() { @Override public void onClick(View view) { num3=Double.parseDouble(num1.getText().toString()); num4=Double.parseDouble(num2.getText().toString()); sum=num3+num4; //txtrslt.setText(Double.toString(sum)); String message = Double.toString(sum); //Intent myIntent; Intent myIntent = new Intent(MainActivity.this,SecondActivity.class); myIntent.putExtra(Send_Result, message); startActivity(myIntent); } }); } }
As we already discussed the result is we are going to display in another page. We need to create a second activity (SecondActivity.java) and a separate layout (result.xml) for displaying the result.
Let’s add another activity by right-click on app in the left-hand panel and select New → Activity → Empty Activity. Give name of the activity as SecondActivity and give layout name as result. Click here to see the graphical view for creating new activity and layout.
This is code for creating a new Intent and send the result of the sum to the second activity by specify the second activity name in the argument list.
Intent myIntent = new Intent(MainActivity.this,SecondActivity.class);
myIntent.putExtra(Send_Result, message);
startActivity(myIntent);
When the control reaches the startActivity(myIntent)method,it calls the second activity and sum shown in the screen.
startActivity(myIntent);
SecondActivity.java
package com.example.nidhi.sumoftwonumbers; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.widget.TextView; /** * Created by nidhi on 12/12/17. */ public class SecondActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Get the view from new_activity.xml setContentView(R.layout.result); // Get the Intent that started this activity and extract the string Intent intent = getIntent(); String message = intent.getStringExtra(MainActivity.Send_Result); // Capture the layout's TextView and set the string as its text TextView textView = findViewById(R.id.reslt); textView.setText(message); } }
Layout for viewing the result.
result.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/reslt" android:layout_width="match_parent" android:layout_height="match_parent" android:text="hai" android:textAlignment="center" android:textSize="50sp" android:layout_marginTop="250sp"/> </LinearLayout>
This is the code for displaying the result.
// Capture the layout’s TextView and set the string as its text
TextView textView = findViewById(R.id.reslt);
textView.setText(message);
As you can see the ‘txtView’ object created in the above code (TextView textView = findViewById(R.id.reslt);) get the reference of TextView created in the result.xml file.
The id of TextView in result.xml layout is reslt.The result of sum of two numbers is displayed using textView.setText(message) method.
Final structure of your android will now looks like
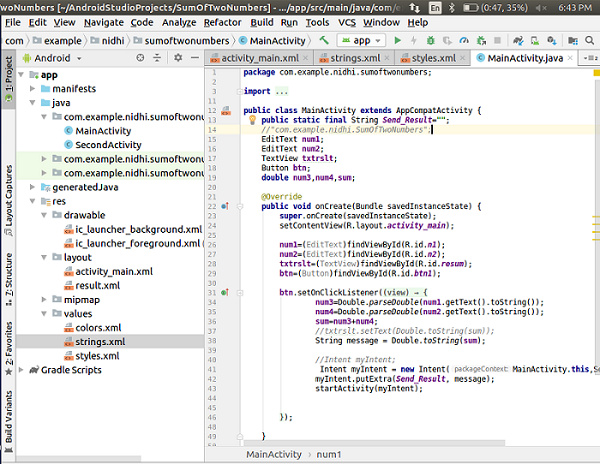
You can now see all the files in the android studio.
The output of the android application for adding two numbers is shown below. When the user input two numbers and click on the button named sum, the result will be shown in another screen.
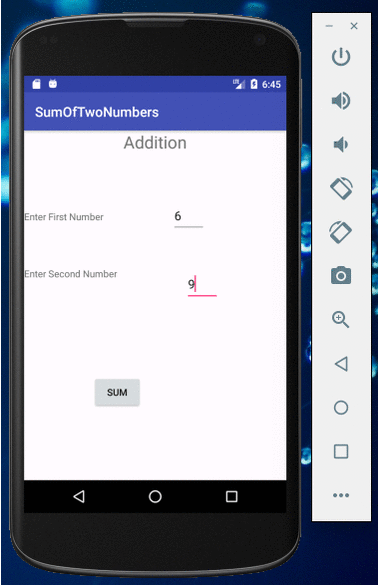
In this very simple and easy tutorial on Android application for adding two numbers, we have learned to develop a simple Android application for adding two numbers. Now all you can use this as basis for implementing a calculator app in android.
I’m mr.gopinath.i would like to become an Android developer.